On Windows Operating System, the PE executables have file extension ending with *.exe and the DLL (Dynamic Link Library) end with *.dll. If you right click the property, and you will find the version number.
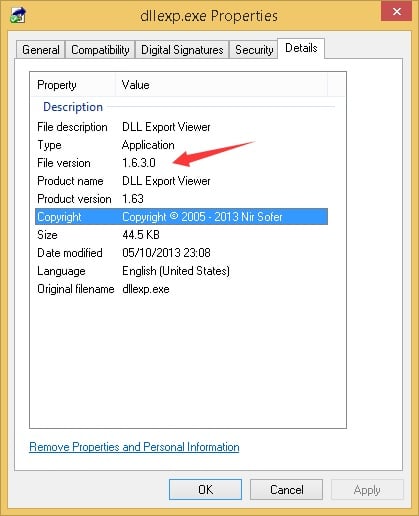
file-version
So, to obtain this string (version) during runtime, we can use Win32 APIs GetFileVersionInfoSizeA, GetFileVersionInfoA and VerQueryValueA
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | int GetFileVersion(const char *filename, char *ver) { DWORD dwHandle, sz = GetFileVersionInfoSizeA( filename, & dwHandle ); if ( 0 == sz ) { return 1; } char *buf = new char[sz]; if ( !GetFileVersionInfoA( filename, dwHandle, sz, & buf[ 0 ] ) ) { delete[] buf; return 2; } VS_FIXEDFILEINFO * pvi; sz = sizeof( VS_FIXEDFILEINFO ); if ( !VerQueryValueA( & buf[ 0 ], "\\", (LPVOID*)&pvi, (unsigned int*)&sz ) ) { delete[] buf; return 3; } sprintf( ver, "%d.%d.%d.%d" , pvi->dwProductVersionMS >> 16 , pvi->dwFileVersionMS & 0xFFFF , pvi->dwFileVersionLS >> 16 , pvi->dwFileVersionLS & 0xFFFF ); delete[] buf; return 0; } |
int GetFileVersion(const char *filename, char *ver) { DWORD dwHandle, sz = GetFileVersionInfoSizeA( filename, & dwHandle ); if ( 0 == sz ) { return 1; } char *buf = new char[sz]; if ( !GetFileVersionInfoA( filename, dwHandle, sz, & buf[ 0 ] ) ) { delete[] buf; return 2; } VS_FIXEDFILEINFO * pvi; sz = sizeof( VS_FIXEDFILEINFO ); if ( !VerQueryValueA( & buf[ 0 ], "\\", (LPVOID*)&pvi, (unsigned int*)&sz ) ) { delete[] buf; return 3; } sprintf( ver, "%d.%d.%d.%d" , pvi->dwProductVersionMS >> 16 , pvi->dwFileVersionMS & 0xFFFF , pvi->dwFileVersionLS >> 16 , pvi->dwFileVersionLS & 0xFFFF ); delete[] buf; return 0; }
Please also note that the GetFileVersionInfoSizeW, GetFileVersionInfoW and VerQueryValueW are the unicode-versions and you should use const wchar_t* filename to pass the unicode file path.
As pointed out, to free the dynamic buffers, we need to use delete [] instead of delete – which will cause memory leaks.
–EOF (The Ultimate Computing & Technology Blog) —
Last Post: How to Decode Hardware ID by VMProtect (using VBScript) ?
Next Post: How to Make Printer a Fax using Epson Connect ?